Overview
The goal of this story is to propose, by default, the configuration of workflow pre-conditions and post-actions at target state globally instead of having to define it for each transition.
For instance I would configure what happens when artifact status becomes "On going", regardless of previous state, rather than having to define what happens when artifact goes from "new" to "On going" + "todo" to "On going" + "review" to "On going", etc.
As pre-conditions and post actions tends to be the same for a given state it reduces the risk of having one specific transition that, un-expectedly behaves diffrently.
The "per transition" configuration would remain possible (at minimum for backward compatibility) but not shown by default.
See live mockup: https://s.codepen.io/enalean/debug/dace752a350bcecdad1003e505bc9992
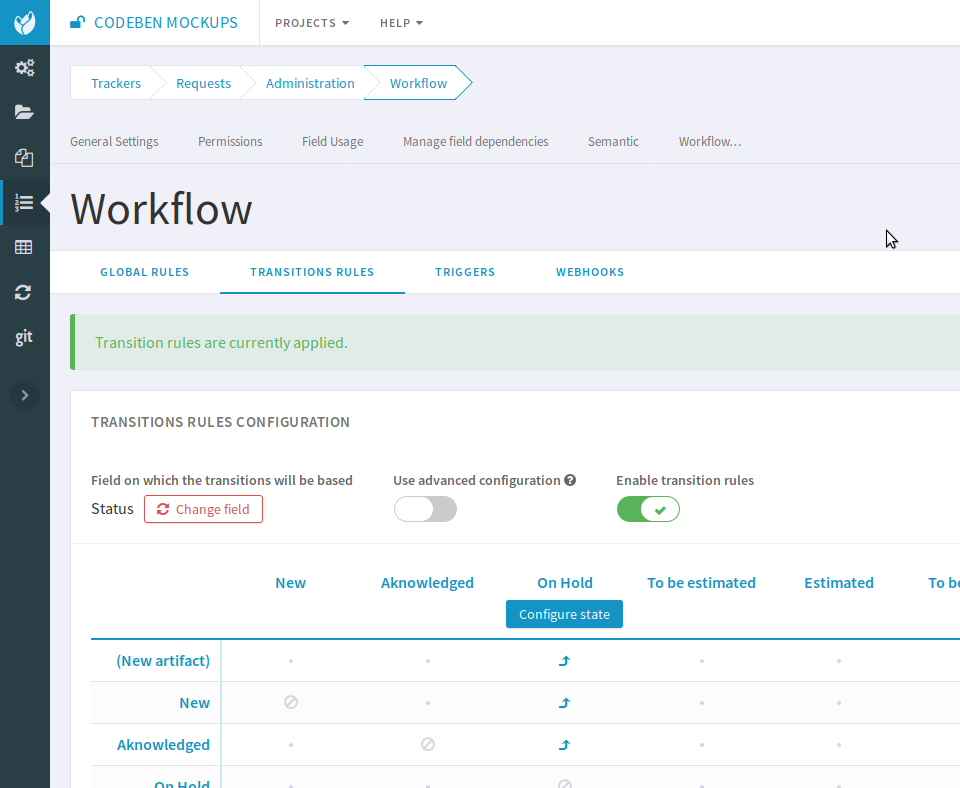
Functional overview
Tracker administrator point of view
The worflow configuration matrix overall design is improved to be easier to read
By default, a click on a transition cell only allow/disallow the transition it self (eg. I allow change from "To do" to "On going") but I don't have a "[details]" link.
The transition configuration screen is accessible by a click on the target state "On going".
A tracker administrator can activate "Advanced mode" where each transition can be configured independently. It's the default mode for all existing trackers. When tracker admin switch from "Simple mode" to "Advanced mode", all configurations are done at transition level (no longer at state level) and switch will copy all configurations (pre-conditions, post-actions that were defined at state level into each defined transition).
On workflow in "Advanced mode", a tracker administrator can activate "Simple mode" (to only have 1 configuration). In this case the first configuration in the target column is selected and applied the whole state. As this operation can break something, a warning message is displayed to ensure tracker admin is sure he wants to continue.
End user point of view
Nothing change. On artifact creation and update, the pre-conditions and post-actions are applied as soon as the target state is reached (given the transition exists in the workflow).
Technical Overview
REST routes
- Get workflow field
- Get configuration mode (advanced or simple)
- Get transition rule activation
- Get all transitions of a tracker
GET /trackers/:id with response content:
{
"workflow": {
"field_id": 0,
"is_used": false,
"transitions": [],
...
},
}
Info: this route already exists
PATCH /trackers/:id with request content:
{ workflow: {
set_transitions_rules: {
field_id: 123
}
}}
Warning: "400 Bad Request" if a field is already defined.
Info: transition rule is disabled by default.
- Remove current workflow field:
PATCH /trackers/:id with request content:
{ workflow: {
delete_transitions_rules: true
}}
Info: this will remove all associated transitions and rules.
- Enable / disable transition rules:
PATCH /trackers/:id with request content:
{ workflow: {
set_transitions_rules: {
is_used: true
}
}}
- Switch between simple and advanced mode:
PATCH /trackers/:id with request content:
{ workflow: {
set_transitions_rules: {
is_advanced: true
}
}}
Info: when switching from advanced mode to simple mode, transitions rules are updated to have same rules by target state.
POST /tracker_workflow_transitions with request content:
{
"tracker_id": int,
"from": int,
"to": int
}
with response content:
{
"id": int,
"uri": string
}
Info: when simple mode, rules of target state is added to this transition
DELETE /tracker_workflow_transitions/:id
- [advanced mode] Get conditions of a transition
GET /tracker_workflow_transitions/:id with response content:
{
"id": int,
"uri": string,
"comment_not_empty": bool,
"fields_not_empty": [ field_id ],
"allowed_ugroups": [ ugroup_id ]
}
Note: tracker_id, from and to are note present here
- [advanced mode] Get all actions of a transition
GET /tracker_workflow_transitions/id/actions with response content:
[
{
"id",
"type",
"settings": {}
},
...
]
- Update rules of a transition
PUT /tracker_workflow_transitions/id/actions with request content:
[
{
"type",
"settings": {}
},
...
]
with response content:
{
"id": int,
"uri": string
}
Info: in simple mode, this will update all other transitions with same target state.
- [simple mode] Get conditions of all transitions to a state
Use "[advanced mode] Get conditions of a transition" with first transition of the state
- [simple mode] Get all actions of all transitions to a state
Use "[advanced mode] Get all actions of a transition" with first transition of the state
- Additional routes to create
OPTIONS /tracker_workflow_transitions
OPTIONS /tracker_workflow_transitions/:id
Frontend
Matrix + modale are made with vue/vuex
Warning: transition from "New artifact" -> XXX some pre-conditions cannot apply (comment not empty). Be carful to handle that properly
On "Simple" mode
The new "simple" mode is actually wrapped around the existing structure and data model where each transition owns pre-conditions and transations. This way there is no modification of workflow checking on the platform, the database layout is not changed, etc. This saves a lot of pain.
However, the backend must ensure the consistency, hence modification of one transition will be propagated to sibling transactions transparently.
When a pre-condition or post-action is applied on 1 transition, the backend force apply on all transitions by truncating existing values and re-creating.
Example, given the following configuration
|from/to | "on going" |
|-----------|--------------------------- |
|"new" | [ |
| | {1, int, {0}}, |
| | {2, jenkins, {"url1"}}, |
| | {3, jenkins, {"url2"}} |
| | ] |
|--------------------------------------- |
|"on going" | X |
|--------------------------------------- |
|"done" | [ |
| | {10, int, {0}}, |
| | {11, jenkins, {"url1"}}, |
| | {12, jenkins, {"url2"}} |
| | ] |
|--------------------------------------- |
When there is a PATCH/PUT on /tracker_workflow_transitions/id/actions to set {2, jenkins, {"url10"}}
This applies to both "new" -> "on going" and "done" -> "on going" transitions and the second one is truncated, hence ids change:
|from/to | "on going" |
|-----------|--------------------------- |
|"new" | [ |
| | {1, int, {0}}, |
| | {2, jenkins, {"url10"}}, |
| | {3, jenkins, {"url2"}} |
| | ] |
|--------------------------------------- |
|"on going" | X |
|--------------------------------------- |
|"done" | [ |
| | {15, int, {0}}, |
| | {16, jenkins, {"url10"}},|
| | {17, jenkins, {"url2"}} |
| | ] |
|--------------------------------------- |
Adding a new field value in Simple mode
When a new field value is added, there is no impact on the transitions themselves. Let say your workflow looks like the following matrix with pre-conditions and post-actions set on Done (materialized with the []).
| Todo | [Done] |
-----|------|--------|
Todo | | x |
-----|------|--------|
Done | | |
-----|------|--------|
When "Archived" state is added, the matrix looks like this
| Todo | [Done] | Archived |
-----|------|--------|----------|
Todo | | x | |
-----|------|--------|----------|
Done | | | |
-----|------|--------|----------|
Arch | | | |
-----|------|--------|----------|
No transition is modified by the addition of this value. However if the transition Arch -> Done is added, the configuration made on [Done] must be applied on Arch -> Done transition.
| Todo | [Done] | Archived |
-----|------|--------|----------|
Todo | | x | |
-----|------|--------|----------|
Done | | | |
-----|------|--------|----------|
Arch | | x | |
-----|------|--------|----------|
Development
The new interface is developed in a new route alongside the existing one. The switch will be done when the new interface will be complete.
In order to not block people with unforeseen use cases and/or work around issues, the switch to the new interface will be progressive. First, there will be a feature flag configuration value to allow users access to the new interface for specific trackers. The old interface will be accessible again after unsetting the configuration value.
Once project admins have added new post actions to their workflow, they will not be able to see, edit or delete them in the old interface. If they want to go back to the old interface, they will first need to clear (delete) their workflow or remove the new post actions with the new interface.
Story split
- Introduce new page (the existing page is left untouched and is still used by default, one should know the new page URL to get access)
- New end-point for WF admin
- The breadcrumbs
- The 2 tab list (2 levels of menu for workflow admin)
- Ability to choose the field on which WF applies (REST PATCH)
- Ability to enable/disable the WF (REST PATCH)
For 4 & 5 see the live mockup here https://s.codepen.io/enalean/debug/5c3599ce2bd94287bb30112569c2d85b#
- Read only matrix
- Display the matrix with CSS helps on big tables
- Display the transitions
- Transitions
- click to activate & desactivate transition
- REST route to save that
- Modale
- Introduce modale to edit transitions. Only pre-conditions can be set
- Modale full
- Modale can be used to configura post-actions
- Introduce a configuration value "feature flag" to allow specific trackers to switch to the new UI. Once set, the "Transitions" button links to the new UI.
- Finalise with "Simple" mode
- Introduce UI to switch from Simple to Advanced mode
- Update REST routes to take into account propagation is "Advanced" mode
- Deprecate old UI